Tracking Your Kraken Balances in MoneyMoney
I wrote a MoneyMoney extension in Lua to track crypto currency balances from a Kraken account.
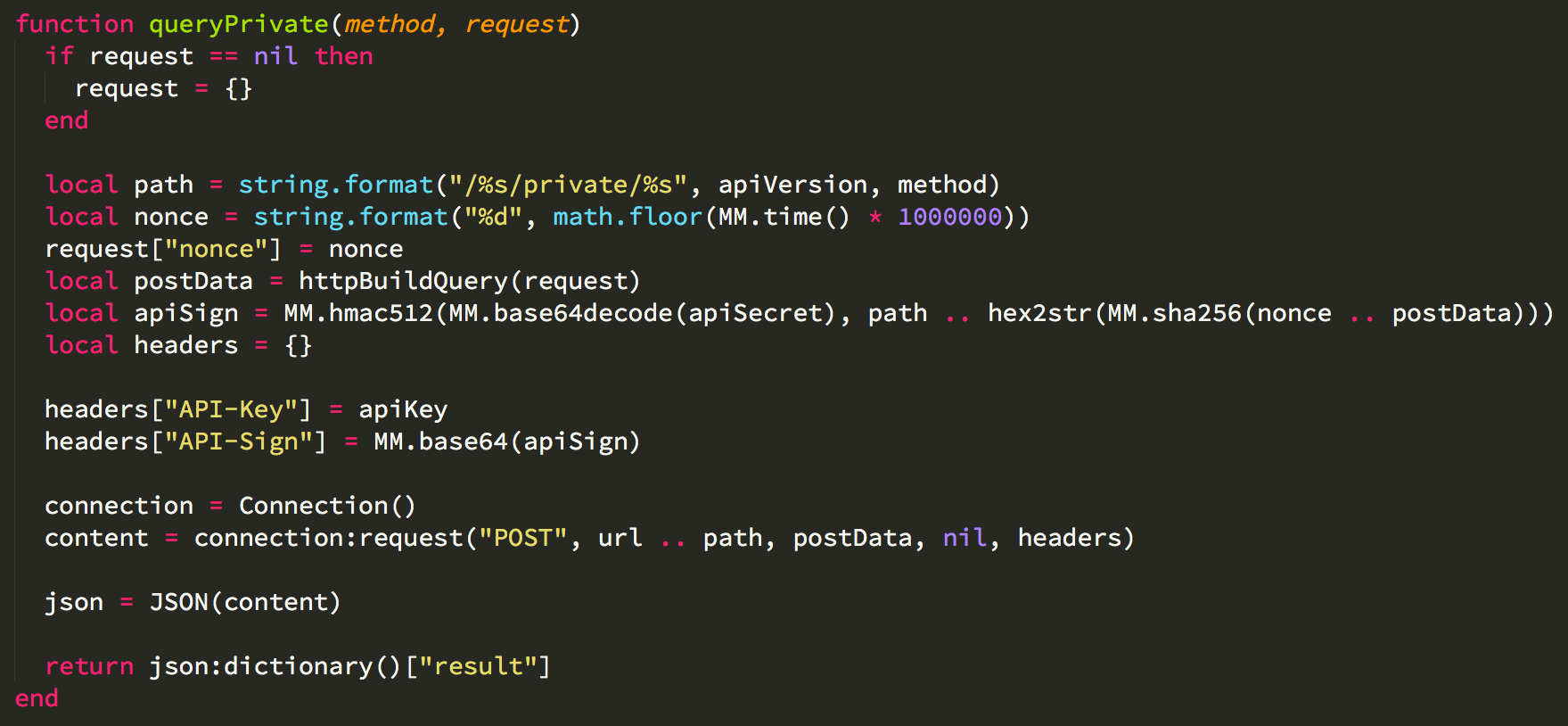
MoneyMoney is a neat banking software for the Mac, mostly targeted to the German market where most banks support the FinTS/HBCI protocol. In addition though, MoneyMoney supports many banks (and similar services such as PayPal) via HTTP-based APIs or—as a last resort—good ol’ web scraping. That’s really great, as it allows you to track virtually all your balances in a single place.
However, what I like most about MoneyMoney are its API and the integrated scripting language: You can roll your own extension with Lua. First, this enables you to write your own import and exports scripts and second—which I find the most interesting—, you can interact with 3rd-party services that aren’t supported out-of-the-box.
So, why not add support for Kraken, my favorite crypto coin exchange?
Not Designed for Crypto Currencies
At first glance, it looks as if you could easily integrate Bitcoin and other crypto currencies. However, at the time of writing, MoneyMoney does not support currencies that require more than two decimal places. So this sadly excludes crypto currencies.
Securities to the Rescue
Luckily, what I intended turned out to be much simpler: I just wanted to see the balances from my Kraken account, multiplied by their current Euro prices. Treating them as securities was an easy and elegant solution to the problem.
Authentication Using HMAC-SHA512
Shortly after starting to write my Kraken extension, I realized that I would need HMAC signing with SHA512 to talk to the Kraken API. That’s something most programming languages have libraries for, but it was lacking in the limited Lua environment I was trapped in. After some unsuccessful googling, I nearly gave up, but I decided to give it a last try and wrote an email to the MoneyMoney support. I asked them if they had any idea how I could achieve my goal—without falling back to web scraping, of course.
The answer was surprising in a very positive way: The creator of MoneyMoney sent me a link to a test version in which he had just implemented a function called MM.hmac512
(and also MM.base64decode
, which I had already done in plain Lua, but gladly replaced right away). Additionally, he sent me a few lines of code that would perform the authorization. Wow!
Only a small change to the code was needed and I was ready to make private requests to the Kraken API:
function queryPrivate(method, request)
if request == nil then
request = {}
end
local path = string.format("/%s/private/%s", apiVersion, method)
local nonce = string.format("%d", math.floor(MM.time() * 1000000))
request["nonce"] = nonce
local postData = httpBuildQuery(request)
local apiSign = MM.hmac512(MM.base64decode(apiSecret), path .. hex2str(MM.sha256(nonce .. postData)))
local headers = {}
headers["API-Key"] = apiKey
headers["API-Sign"] = MM.base64(apiSign)
connection = Connection()
content = connection:request("POST", url .. path, postData, nil, headers)
json = JSON(content)
return json:dictionary()["result"]
end
Getting it to Work
Knowing Lua from Rack Extension development (those are virtual instruments for Propellerhead’s Reason), the rest was pretty straight-forward. Shortly after, I could see the balances from my test account:
Great success!
Download
You can find my extension on the MoneyMoney Extensions page or on GitHub (along with the source code).
Updates
- Aug 15, 2017: Looks like my work inspired others to write their own crypto currency extensions. Check out the MoneyMoney Extensions page—there are now extensions to support privately-held keys, as well as other exchanges. Yay!